Svelte and Tailwind for Chrome Extension
A step-by-step guide on how to create a Chrome Extension using Svelte and Tailwind CSS
Thursday, February 16, 2023
Introduction
This article will show you how to create a Chrome Extension using hot frameworks such as Svelte and Tailwind CSS. This will also use the very popular Vite as the build tool.
Here are some definitions of the tech choices according to ChatGPT.
What is Svelte?
Svelte is a JavaScript framework that compiles your code into efficient JavaScript that surgically updates the DOM. It is a compiler that converts your code into a more efficient version of itself.
What is a Chrome Extension?
A Chrome extension is a software program that extends the functionality of Google Chrome. It modifies the browser's behavior and adds new features.
What is Tailwind CSS?
TailwindCSS is a utility-first CSS framework for rapidly building custom user interfaces. It is a CSS framework that provides a set of pre-built classes that can be used to style your HTML elements.
NOTE: This might be an overcomplicated setup for a simple Chrome Extension. On larger projects, we will see the benefits of using the mentioned tools to improve the development process.
Setting up the project
Make sure you have node.js v16.x or greater
Install Svelte
Initialize the project using vite
- Select a project name
- Select
Svelte
- Select
TypeScript
- Follow the output instruction
Open the URL in a browser, and you should see the following result.
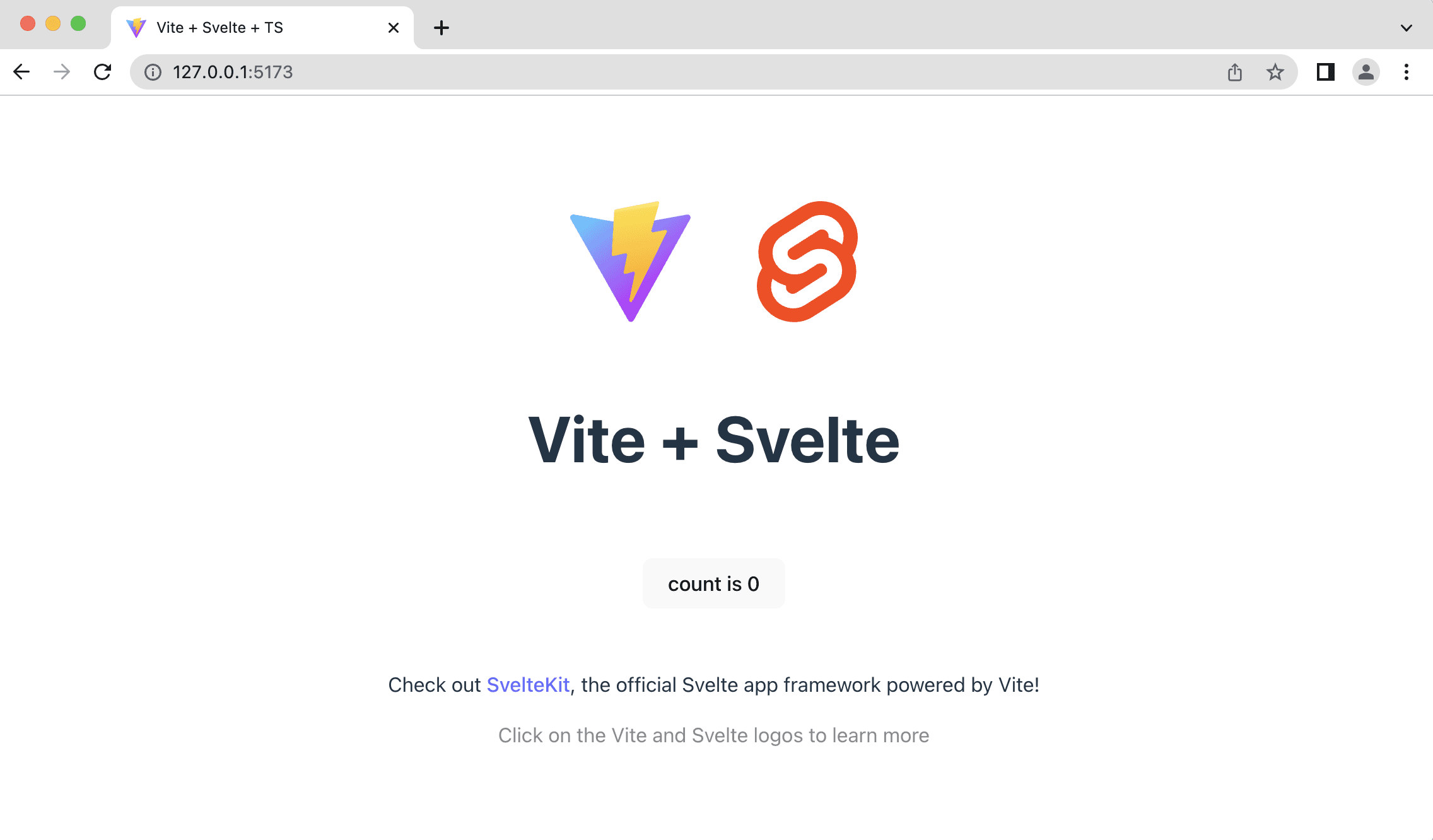
Install Tailwind
- Install Tailwind dependencies
- Initialize default Tailwind configuration
- Make sure to enable use of POSTCSS in
style
blocks
svelte.config.js12345
- Configure
content
paths
tailwind.config.js1234567
- Replace the content of
src/app.css
with the following
src/app.css1234567891011
Testing Tailwind integration
Add tailwind styles
Add any styles that will be obvious when the app is running.
.html12
And you should see the following result. We have a heading with dark-red background and light-red text.
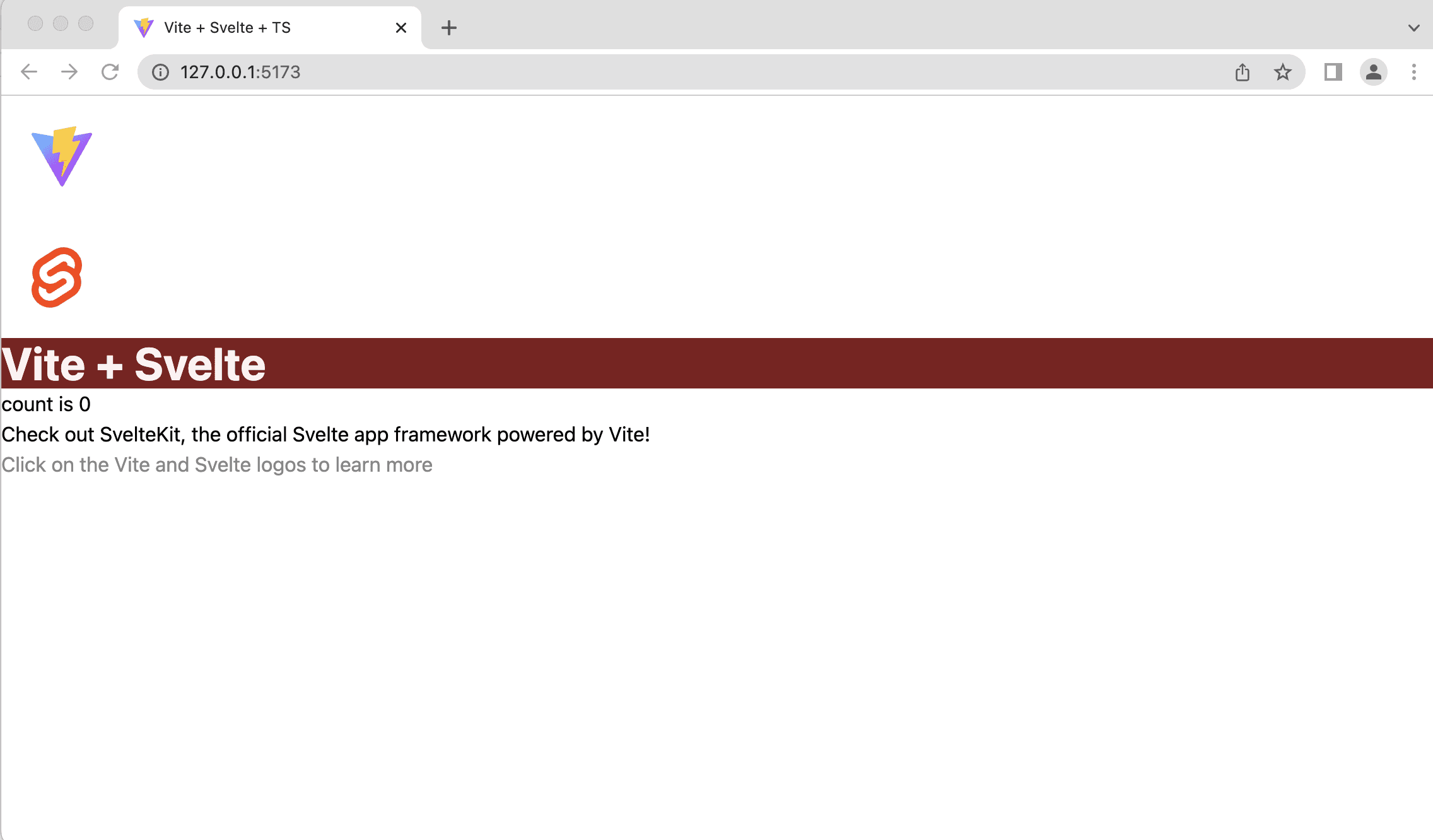
Remove unused files
Now that we verify that the app is working as expected, we can remove the unused files.
- Delete
src/App.svelte
- Delete
src/main.ts
- Delete
index.html
- Delete
src/assets/svelte.png
- Delete
src/lib
folder
Create a very basic chrome extension
Install Chrome Extension Library for vite
Instead of complicating the setup, we will crxjs to help us simplify the development process.
Create a manifest file
The manifest file contains the necessary information for the browser to load the extension. For more information, check out the Chrome Extension Manifest
manifest.json12345678910
NOTE: The
storage
permission is added because we will use it later.
Add the plugin to vite.config.js
vite.config.js12345678
Optional configuration for TypeScript
Update TypeScript configuration files
For some reason, scripts work as expected until these options are added
tsconfig.json123456
tsconfig.node.json12345678
Improve Chrome Plugin TypeScript support
To get better TypeScript support, install the chrome type definitions
Create the content of the popup plugin
Creating the content of the plugin is as simple as creating a web typical page. We still use HTML, JavaScript, and CSS. The obvious difference is where we can view the content.
The markup below is the content that will be displayed when the popup extension is opened.
src/popup/index.html1234567891011121314151617
It is important to match the absolute file path with the one in the manifest file.
.json123
Create the script file to load CSS(and other stuff)
To make sure Tailwind styles are processed, don't forget to import the CSS file in the script file.
src/popup/index.ts1
Again, it is important to match the relative file path in the script tag
Build and load the extension
- Run
npm run dev
ornpm run build
- Open the chrome extension page by typing
chrome://extensions
in the address bar - Enable the developer mode
- Click on the
Load unpacked
button - Select the
dist
folder - Open the extension menu; then, click the loaded extension
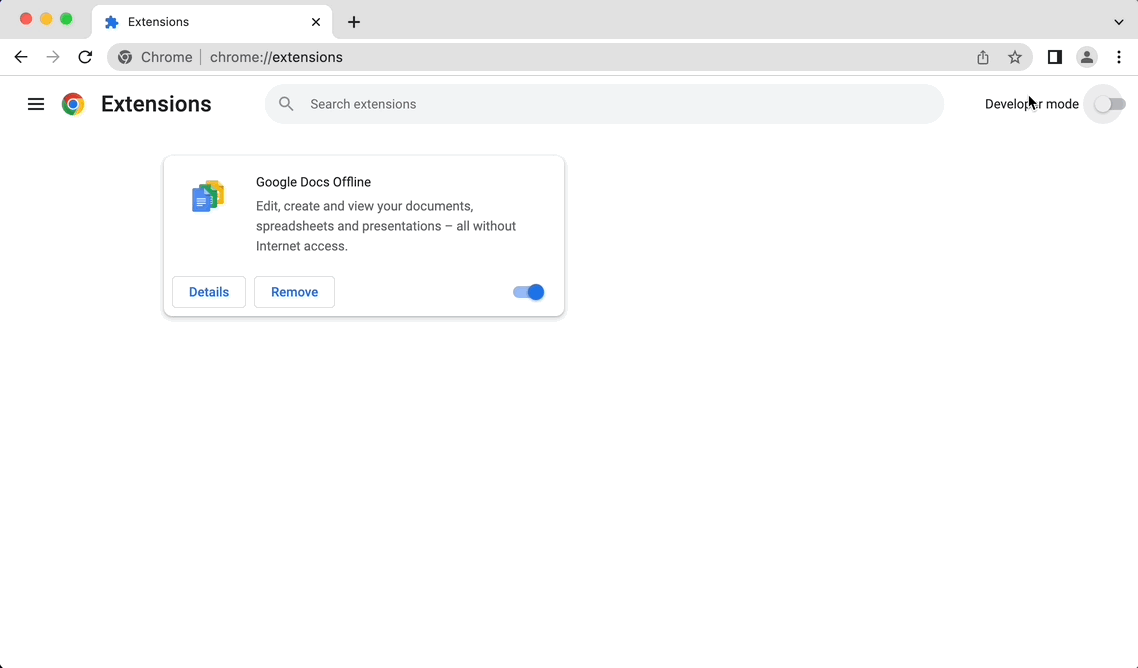
Add interaction using Svelte
Now let's make the extension interactive. We will create a counter that will be saved in the browser storage.
Create a reusable counter component
Create a simple and reusable counter component that can be used in any part of the application.
src/components/Counter.svelte12345678910111213141516171819202122232425262728293031323334353637383940414243444546
If the code above does not make any sense, check out the Svelte tutorial
Update the popup script
src/popup/index.ts123456789101112
Remove unnecessary stuff in the HTML file
src/popup/index.html1234567891011
Re-test the extension
There should be no need to rebuild the app because crxjs has HMR enabled by default. If not, just reload the extension.
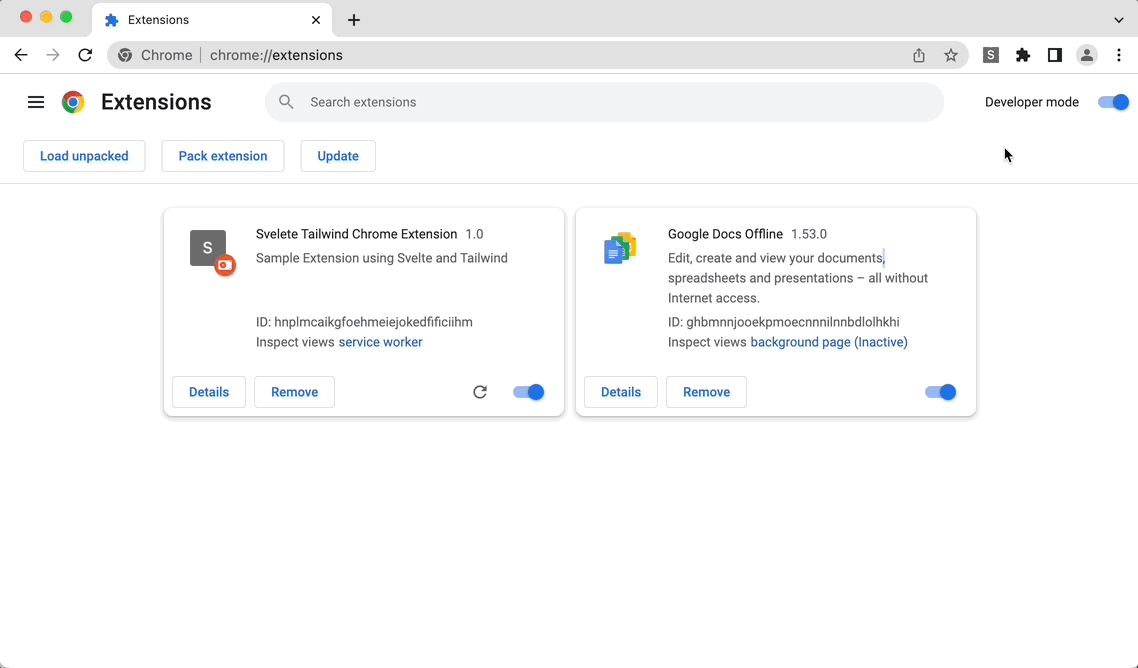
Create a New Tab extension
A new tab extension is an extension that replaces the default new tab page with a custom one. Creating a new tab extension is almost the same as creating a popup extension. The only difference is the manifest file.
manifest.json123456
Copy-paste the popup HTML file to the new tab HTML file
src/new-tab/index.html1234567891011
Copy-paste the popup JS file to the new tab JS file
src/popup/index.ts123456789101112
Re-test the extension
Just like magic, the new tab extension is working.
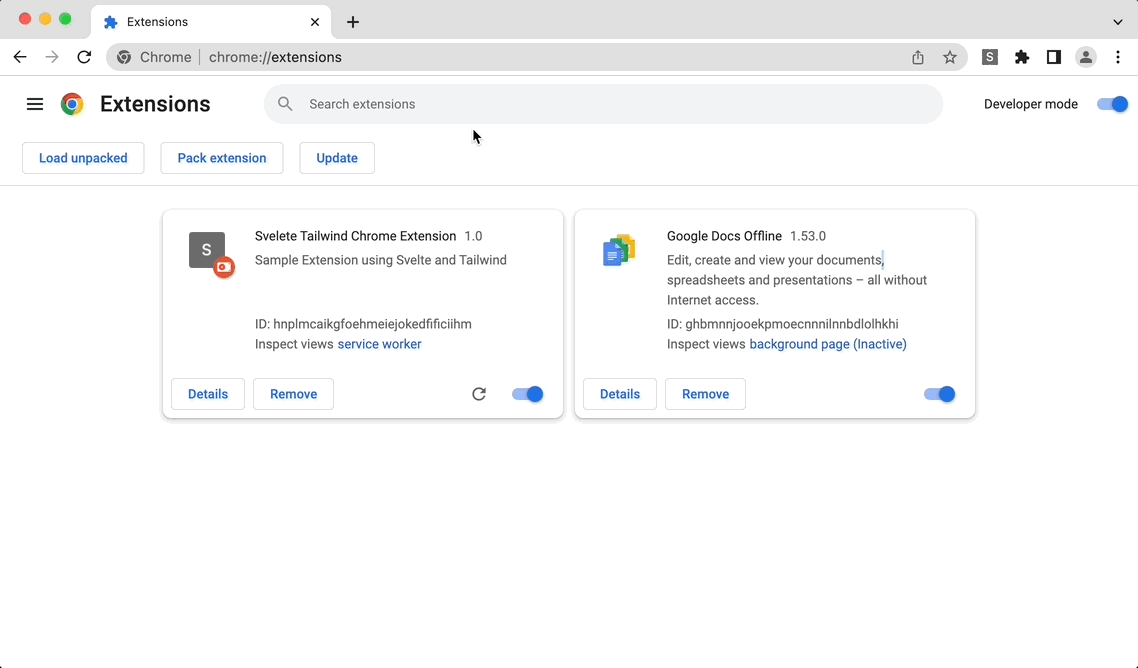
(BONUS) Awesome HMR support
By default, crxjs has HMR enabled. This is a big productivity boost for developers!!!
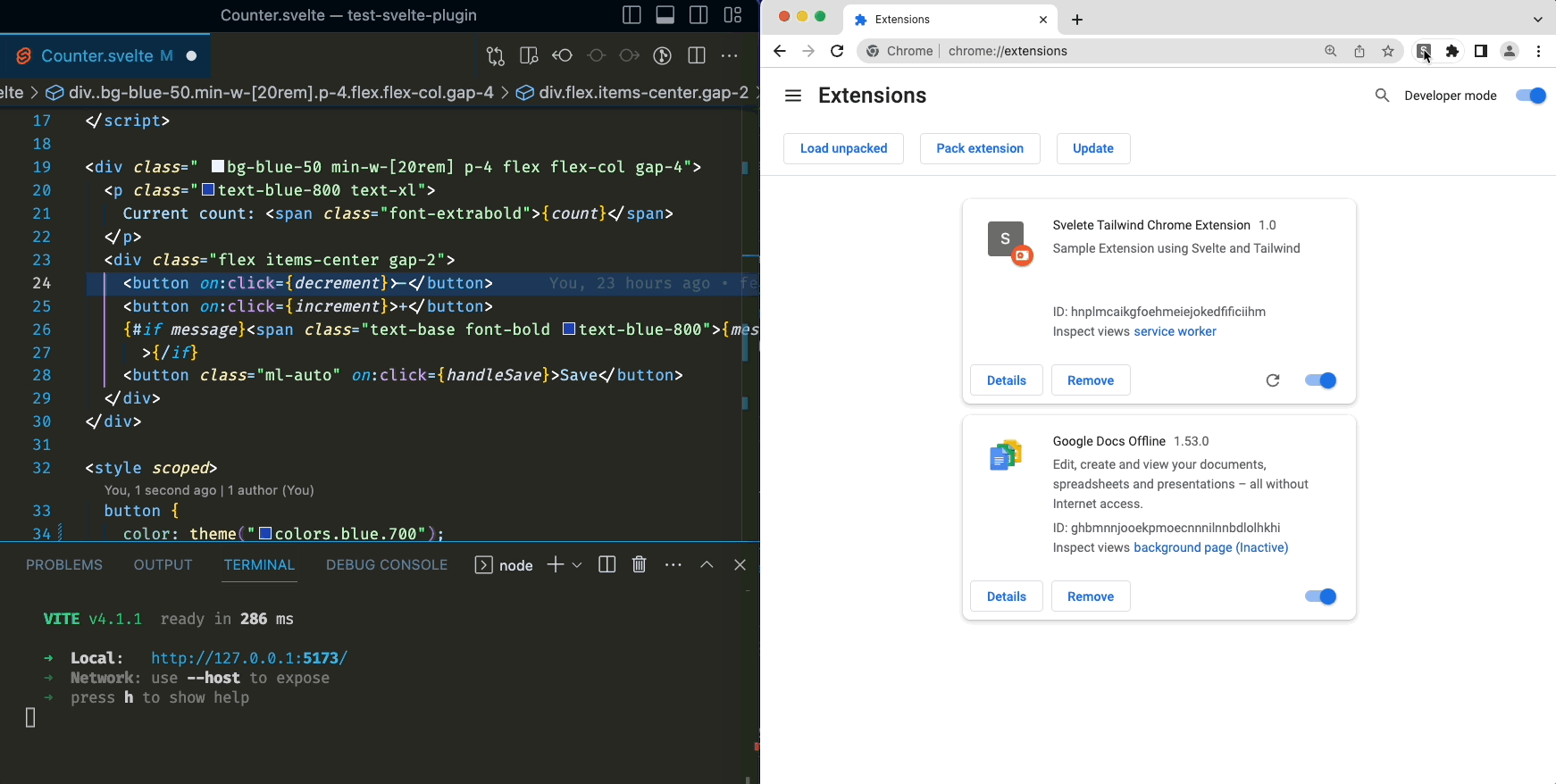
Repository
Check the source code here
What's next
- ✅ Add an options page
- ✅ Sync plugin contents without reloading
- [ ] Add a content script
- [ ] Add a background script
- [ ] Add a dev tools page
- [ ] Deploying the extension